Actual Execution of program starts when it loads on main memory (RAM) . Memory assigned in the RAM can be divided into the four segments .
- First segment use to store instructions
- Second segment of the memory is use to store Global/static variable (these variables are not declared under any function such as main).
- Stack is use to store the variable under the function’s stack frame. Function’s frame is created in RAM as soon as function called in the program. Once the execution of function completed function’s stack frame automatically get deleted and all the variable defined in function’s stack frame get erased.
- The heap is a large pool of memory that can be used dynamically – it is also known as the “free store”. This is memory that is not automatically managed – you have to explicitly allocate (using functions such as malloc), and deallocate (e.g. free) the memory
Note: Unlike Heap amount of memory allocated in the first three sections (instruction, Global/static and Stack) can not be changed.
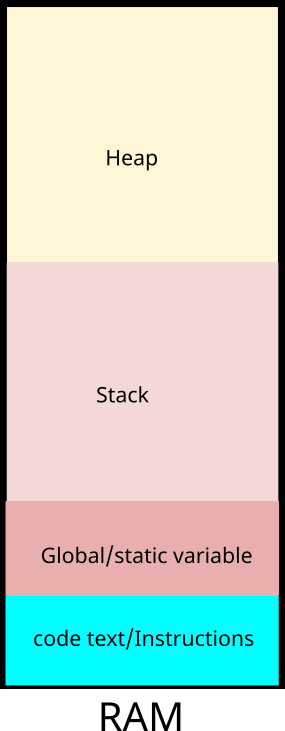
#include
int sum;
int f1(int x, int y)
{
int add =x+y;
return add;
}
int main()
{
int a =2, b=3;
sum = f1(a,b);
printf("Sum of %d and %d is %d \n", a,b,sum);
return 0;
}